How to Integrate Midjourney API in Your Projects
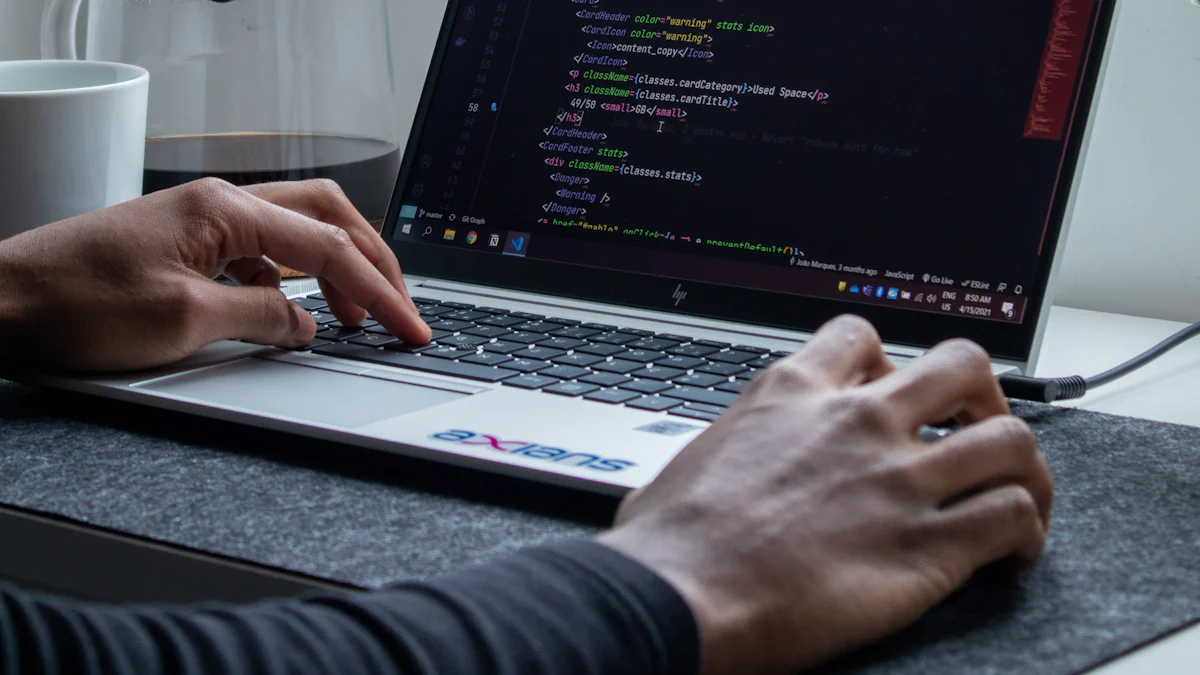
The Midjourney API offers a powerful tool for developers to create high-quality images and visual content programmatically. Integrating APIs into projects has become essential in modern development workflows. APIs provide functionalities like rapid prototyping, automated testing, and data integration. Businesses invest heavily in APIs because they accelerate product development and ensure scalability. The Midjourney API stands out by enabling developers to leverage AI drawing capabilities, enhancing applications with stunning visuals. Although there is no Midjourney official API yet, developers can still gain Midjourney API access through unofficial clients. While there isn’t a Midjourney free API available, the Midjourney API cost is justified by the high-quality output it provides.
Understanding the Midjourney API
What is the Midjourney API?
The Midjourney API offers developers a robust tool for creating high-quality images and visual content. This API provides functionalities that support various stages of product development. These stages include ideation, design, testing, and deployment.
Key Features and Benefits
The Midjourney API includes several key features:
- Rapid Prototyping: Quickly create mockups and prototypes.
- Automated Testing: Streamline testing processes with automated tools.
- Data Integration: Seamlessly integrate data from different sources.
- Scalability: Ensure your application can handle increased loads.
These features make the Midjourney API invaluable for developers. The API enhances productivity and ensures high-quality output.
Use Cases in Various Projects
The Midjourney API can be used in multiple scenarios. Here are some examples:
- Enhance product listings with realistic mockups.
- Create eye-catching graphics for social media marketing campaigns.
- Generate unique custom illustrations for branding purposes.
- Implement real-time language translation in applications.
- Automate content generation for blogs or websites.
- Perform intelligent data analysis to improve user experience.
These use cases demonstrate the versatility and power of the Midjourney API.
Prerequisites for Integration
Before integrating the Midjourney API, you need to prepare your development environment. This preparation ensures a smooth integration process.
Required Tools and Software
To get started, you will need:
- A development environment (e.g., Visual Studio Code, PyCharm).
- An HTTP client (e.g., Postman, cURL).
- Programming languages such as Python, JavaScript, or PHP.
- Access to the Midjourney API documentation.
These tools and software are essential for working with the Midjourney API.
Setting Up Your Development Environment
Follow these steps to set up your environment:
- Install a Code Editor: Download and install a code editor like Visual Studio Code.
- Set Up an HTTP Client: Install Postman or cURL for testing API requests.
- Install Necessary Libraries: Use package managers like npm or pip to install required libraries.
- Obtain API Keys: Sign up on APIFRAME to get your Midjourney API keys.
Setting up your environment correctly will help you avoid common issues during integration.
Setting Up the Midjourney API
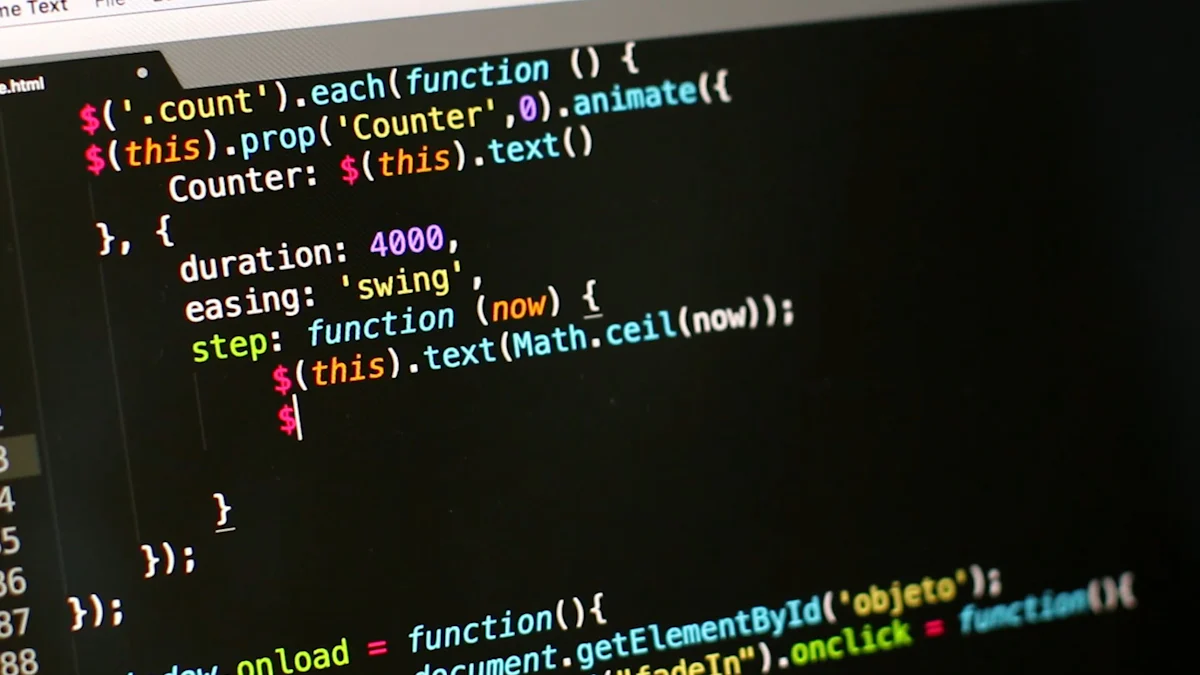
Creating an Account and Getting API Keys
Step-by-step account creation
- Visit the Official Platform: Navigate to the official MidJourney platform.
- Sign Up: Click on the sign-up button. Enter your email address and create a password.
- Verify Email: Check your email for a verification link. Click on the link to verify your account.
- Complete Profile: Fill in any additional profile information requested by the platform.
Creating an account provides access to the necessary tools for integrating the MidJourney API into your projects.
How to obtain API keys
- Log In: Log in to your newly created account on the MidJourney platform.
- Navigate to API Section: Find the API section in your account dashboard.
- Generate API Key: Click on the button to generate your unique API key.
- Copy API Key: Copy the API key and store it securely. You will need this key to authenticate your requests.
Obtaining an API key is essential for making authenticated requests to the MidJourney API.
Installing Necessary Libraries
Installation commands for different environments
To integrate the MidJourney API, you need to install specific libraries. Follow these commands based on your development environment:
- Python:
pip install requests
- JavaScript (Node.js):
npm install axios
- PHP:
composer require guzzlehttp/guzzle
Installing these libraries ensures that your development environment can interact with the MidJourney API.
Verifying the installation
- Create a Test Script: Write a simple script to test the library installation.
- Run the Script: Execute the script in your development environment.
- Check for Errors: Ensure there are no errors during execution.
For example, in Python, create a file named test.py
:
import requests
response = requests.get('https://api.example.com/test')
print(response.status_code)
Run the script:
python test.py
Successful execution confirms that the necessary libraries are installed correctly. This step ensures that your environment is ready for further integration with the MidJourney API.
Integrating the Midjourney API into Your Project
Basic Integration Steps
Writing the initial code
Start by setting up a new project in your preferred programming language. Create a new file where you will write the initial code. Import the necessary libraries to interact with the Midjourney API.
For example, in Python:
import requests
api_key = 'your_api_key_here'
endpoint = 'https://api.midjourney.com/v1/generate'
headers = {
'Authorization': f'Bearer {api_key}',
'Content-Type': 'application/json'
}
data = {
'prompt': 'Create a high-quality image of a sunset over mountains'
}
response = requests.post(endpoint, headers=headers, json=data)
print(response.json())
This code sends a request to the Midjourney API to generate an image based on a given prompt. Replace 'your_api_key_here'
with your actual API key.
Testing the integration
Run the script in your development environment. Verify that the response from the API contains the expected data. Check for any errors or issues in the response.
For example, in Python:
python your_script_name.py
Ensure that the response includes a URL to the generated image or other relevant data. If the response contains errors, review the error messages and adjust the code accordingly.
Advanced Integration Techniques
Handling API responses
Properly handling API responses ensures that your application can process the data effectively. Parse the JSON response to extract the necessary information.
For example, in Python:
response_data = response.json()
if response.status_code == 200:
image_url = response_data['image_url']
print(f"Generated Image URL: {image_url}")
else:
print(f"Error: {response_data['message']}")
This code checks the status code of the response and extracts the image URL if the request was successful. Handle different status codes to provide meaningful feedback to users.
Error handling and debugging
Implement robust error handling to manage potential issues during API integration. Use try-except blocks to catch exceptions and log errors for debugging.
For example, in Python:
try:
response = requests.post(endpoint, headers=headers, json=data)
response.raise_for_status()
response_data = response.json()
image_url = response_data['image_url']
print(f"Generated Image URL: {image_url}")
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"Other error occurred: {err}")
This code catches HTTP errors and other exceptions, providing detailed error messages. Proper error handling improves the reliability of your application.
Practical Examples and Use Cases
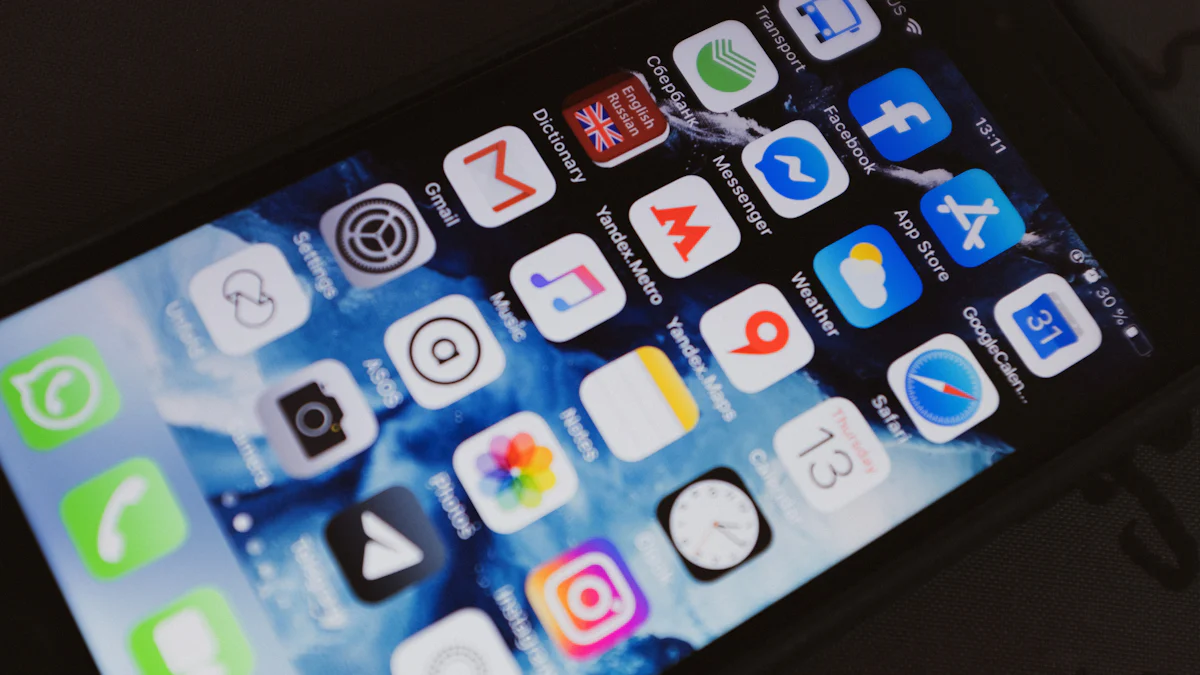
Example 1: Integrating with a Web Application
Step-by-step guide
- Set Up Your Project: Create a new web application project using your preferred framework (e.g., React, Angular).
- Install Required Libraries: Use npm to install Axios for making HTTP requests.
npm install axios
- Create API Service: Create a new file named
apiService.js
to handle API requests.import axios from 'axios'; const apiKey = 'your_api_key_here'; const endpoint = 'https://api.midjourney.com/v1/generate'; export const generateImage = async (prompt) => { try { const response = await axios.post(endpoint, { prompt: prompt }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data; } catch (error) { console.error('Error generating image:', error); throw error; } };
- Integrate API in Component: Use the API service in your component to generate images.
import React, { useState } from 'react'; import { generateImage } from './apiService'; const ImageGenerator = () => { const [prompt, setPrompt] = useState(''); const [imageUrl, setImageUrl] = useState(''); const handleGenerate = async () => { try { const data = await generateImage(prompt); setImageUrl(data.image_url); } catch (error) { console.error('Error:', error); } }; return ( <div> <input type="text" value={prompt} onChange={(e) => setPrompt(e.target.value)} placeholder="Enter prompt" /> <button onClick={handleGenerate}>Generate Image</button> {imageUrl && <img src={imageUrl} alt="Generated" />} </div> ); }; export default ImageGenerator;
Code snippets and explanations
The code above demonstrates how to integrate the MidJourney API into a web application. The apiService.js
file handles API requests using Axios. The ImageGenerator
component allows users to input a prompt and generate an image.
Example 2: Integrating with a Mobile App
Step-by-step guide
- Set Up Your Project: Create a new mobile app project using React Native.
- Install Required Libraries: Use npm to install Axios for making HTTP requests.
npm install axios
- Create API Service: Create a new file named
apiService.js
to handle API requests.import axios from 'axios'; const apiKey = 'your_api_key_here'; const endpoint = 'https://api.midjourney.com/v1/generate'; export const generateImage = async (prompt) => { try { const response = await axios.post(endpoint, { prompt: prompt }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }); return response.data; } catch (error) { console.error('Error generating image:', error); throw error; } };
- Integrate API in Component: Use the API service in your component to generate images.
import React, { useState } from 'react'; import { View, TextInput, Button, Image, StyleSheet } from 'react-native'; import { generateImage } from './apiService'; const ImageGenerator = () => { const [prompt, setPrompt] = useState(''); const [imageUrl, setImageUrl] = useState(''); const handleGenerate = async () => { try { const data = await generateImage(prompt); setImageUrl(data.image_url); } catch (error) { console.error('Error:', error); } }; return ( <View style={styles.container}> <TextInput style={styles.input} value={prompt} onChangeText={setPrompt} placeholder="Enter prompt" /> <Button title="Generate Image" onPress={handleGenerate} /> {imageUrl && <Image source={{ uri: imageUrl }} style={styles.image} />} </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', padding: 16, }, input: { height: 40, borderColor: 'gray', borderWidth: 1, marginBottom: 12, paddingHorizontal: 8, width: '100%', }, image: { width: 200, height: 200, marginTop: 12, }, }); export default ImageGenerator;
Code snippets and explanations
The code above demonstrates how to integrate the MidJourney API into a mobile app. The apiService.js
file handles API requests using Axios. The ImageGenerator
component allows users to input a prompt and generate an image.
Troubleshooting Common Issues
Common Errors and Their Solutions
Authentication issues
Authentication issues often arise when integrating the Midjourney API. Ensure that the API key used matches the one provided by the platform. Double-check the key for any typos or missing characters. Store the API key securely to prevent unauthorized access.
If authentication fails, verify that the API key has not expired. Some platforms issue keys with limited lifespans. Renew the key if necessary. Also, confirm that the API endpoint URL is correct. Incorrect URLs can lead to failed authentication attempts.
API request errors
API request errors can disrupt the integration process. Common causes include incorrect request formats and missing parameters. Always refer to the API documentation for the correct request structure. Ensure that all required parameters are included in the request.
Network issues can also cause request errors. Check your internet connection and ensure that the server hosting the API is operational. Use tools like Postman to test API requests and identify potential issues.
Best Practices for Smooth Integration
Tips for maintaining API connections
Maintaining stable API connections ensures smooth operation. Use retry mechanisms to handle temporary network failures. Implement exponential backoff strategies to avoid overwhelming the server with repeated requests.
Monitor the API usage to stay within rate limits. Exceeding rate limits can result in throttling or temporary bans. Use logging to track API requests and responses. Logs help identify patterns and potential issues.
Performance optimization
Optimize performance by minimizing the number of API calls. Batch multiple requests into a single call when possible. Reduce the payload size to improve response times. Compress data before sending it to the server.
Cache frequently accessed data to reduce the load on the API. Use local storage or in-memory caching solutions. Regularly review and update the integration code to incorporate performance improvements and best practices.
You have now explored the essentials of integrating the Midjourney API into your projects. The Midjourney API offers powerful tools for generating high-quality images and visual content. Experiment with the API to unlock its full potential. Your applications can benefit from enhanced visuals and improved user engagement.
“Our social media application has been transformed thanks to your API. It helped us to generate very nice images just from text inputs. Our user engagement went up a lot!”
Feel free to share feedback or ask questions. Your insights can help improve future integrations. Happy coding!